ブラウザの[戻る]を押してね!
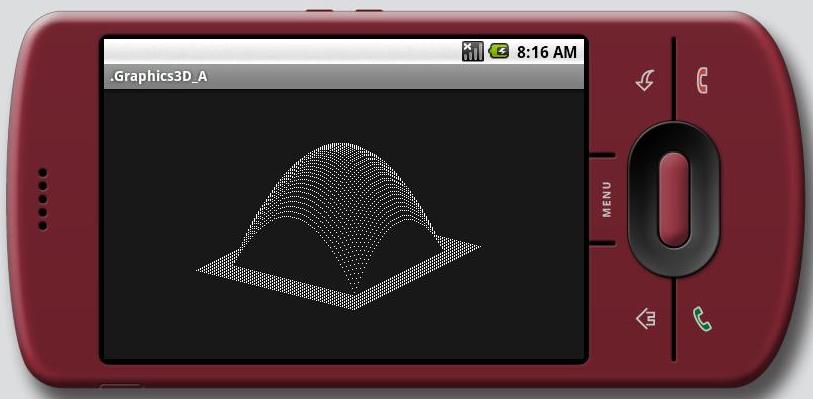
< Android 版 Java でのソースプログラム内容 >
/*
3次元グラフィックス表示プログラム A (Android 用バージョン)
(とりあえず、図形をひとつだけ表示してみたもの)
*/
package test.graphics3D_A;
import android.app.Activity;
import android.os.Bundle;
public class Graphics3D_A extends Activity {
Graphics3DView graphics3DView;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
graphics3DView = new Graphics3DView( this );
setContentView(graphics3DView);
}
}
package test.graphics3D_A;
import android.content.Context;
import android.graphics.Canvas;
import android.graphics.Paint;
import android.view.View;
public class Graphics3DView extends View {
int icnt; // ループカウンタなど用のワーク変数
int x3d, y3d, z; // 3次元座標軸上でのx、y、zの値
int x2d, y2d; // 2次元座標軸上でのx、yの値
int max[] = new int[400]; // 最大値格納用配列
int min[] = new int[400]; // 最小値格納用配列
Paint linePaint; // 線の描画方法
public Graphics3DView(Context context) {
super(context);
// 最大値/最小値配列の初期化
for ( icnt=0; icnt<400; icnt++ ) {
max[icnt] = 0;
min[icnt] = 400;
}
// 線の描画方法を指定する
linePaint = new Paint();
linePaint.setStyle(Paint.Style.STROKE);
linePaint.setColor(0xffffffff);
}
protected void onDraw(Canvas canvas) {
// 3次元図形表示処理
for ( y3d=0; y3d<128; y3d +=2 ) {
for ( x3d=0; x3d<160; x3d +=2 ) {
// Z値算出処理(任意の関数 z=f(x3d, y3d) に置き換え可能)
if (((x3d >= 20) && (x3d <= 140)) && ((y3d >= 20) && (y3d <= 108))) {
z = ((x3d - 80) * (x3d - 80)) / 60 + ((y3d - 64) * (y3d - 64)) / 40;
} else {
z = 110;
}
// 3次元→2次元座標変換処理
x2d = 250 - (x3d - y3d);
y2d = z + 210 - ((x3d / 4) + (y3d / 2) + 100);
// 陰線処理(最大値/最小値判定による)
if ((y2d >= max[x2d]) || (y2d <= min[x2d])) {
if (y2d >= max[x2d]) {
max[x2d] = y2d;
}
if (y2d <= min[x2d]) {
min[x2d] = y2d;
}
if ((y2d >= 0) && (y2d < 500)) {
// 直線を用いて点を描画する
canvas.drawLine(x2d, y2d, x2d+1, y2d, linePaint);
}
}
}
}
}
}